# 3.4 Alternative payment methods
# 3.4.1 Alternative wallets
- ApplePay
- GooglePay
- PayPal
- AmazonPay
- MasterPass
- Visa Checkout
- Amex Checkout
Alternative payment methods that act as a wallet, and enables the users to add a credit card to the wallet, and re-use in the future. The flow is similar to a credit card token flow.
ApplePay is a exception, and the ApplePay token is not added to the wallet. [[using ApplePay]](Alternative Payment methods.md#342-using-applepay)
# How to get the token
Listening to the
afterSelectCreditCard
event.
[Read more]Requesting the credit card token explicitly using
mycheckWallet.getCardToken()
.
[Read more]
# How to charge the token
To charge the token, you can use our Billing API,
using AUTH + CHARGE methods, or SALE.
[Read more]
# 3.4.2 Using ApplePay
In order to test and accept Apple Pay on the web, you'll need to generate a Payment Processing Certificate and register any domains you plan to use with Apple. You can do this through the Apple Pay Developers Panel using the instructions in the following link: https://developer.apple.com/videos/play/tutorials/configuring-your-developer-account-for-apple-pay/ (opens new window)
In order to use ApplePay, you need to set up the amount and currency That the user will need to approve
mycheckWallet.setPayment({"currency":"USD","amount":"1.00"});
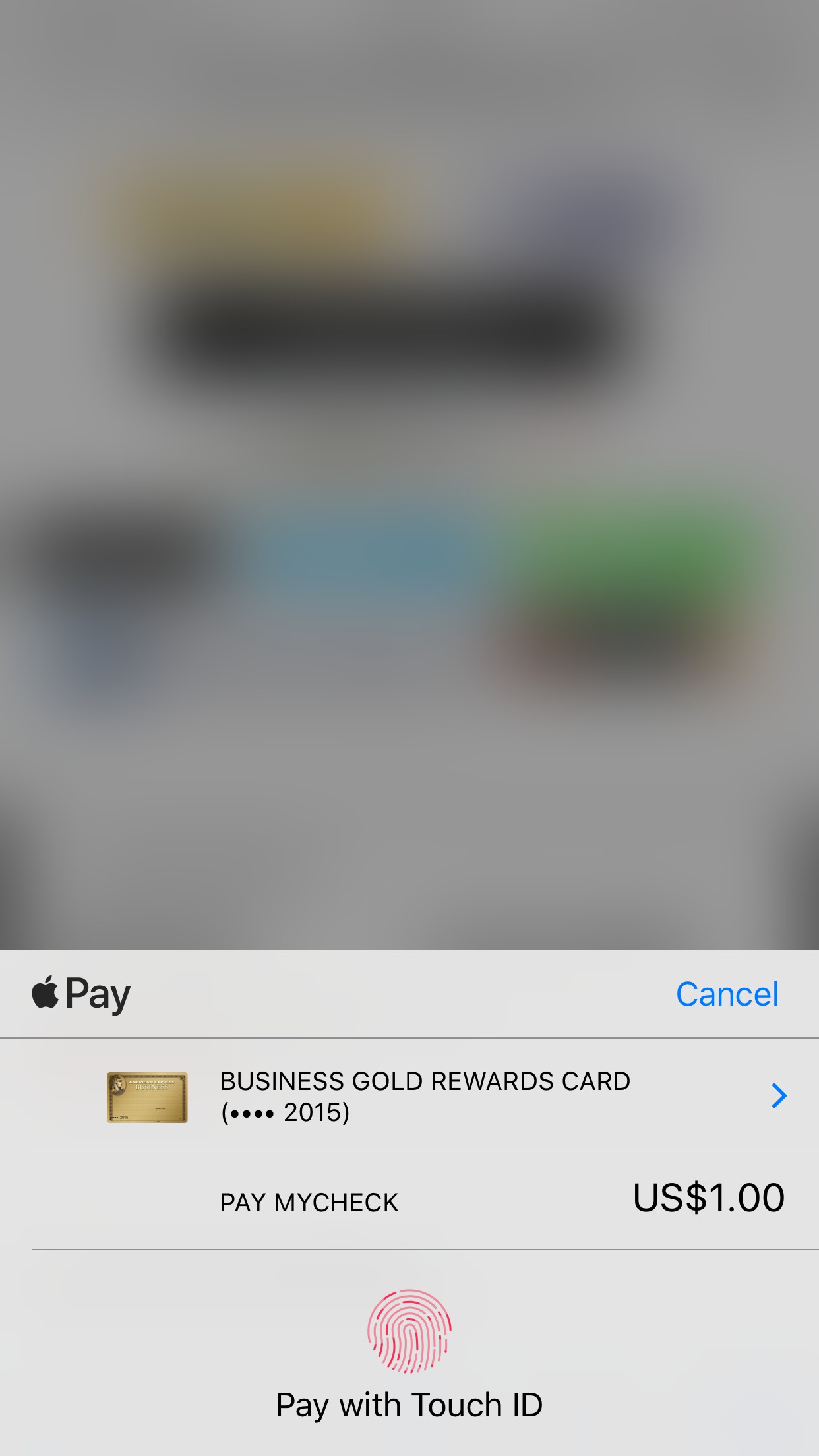
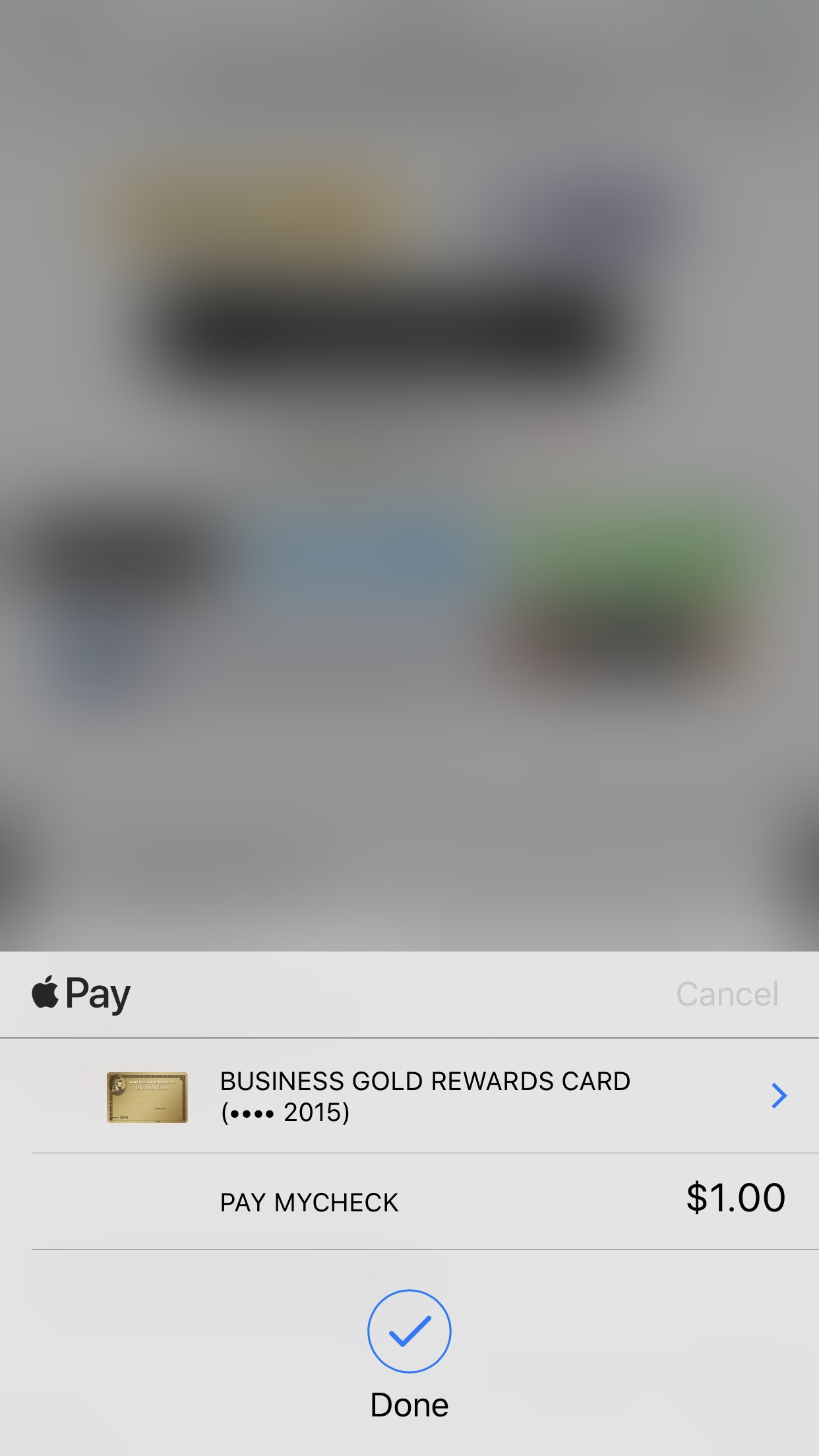
After the payment flow completed, you can use the same function as in the previous section (under "How to get the token") to recieve the credit card token, and use AUTH+CHARGE / SALE methods to complete the payment.
# 3.4.2.1 Using PayPal
Set up and configure the following accounts: PayPal - A valid PayPal and PayPal business test account.
Link your PayPal account(s) to your Shiji merchant account by adding it in Shiji dashboard:
- privateKey
- merchantId
- publicKey
[To enable or disable it in Wallet]
# 3.4.3 Instant payments
- AliPay
- WeChatPay
- iDeal
Alternative payment methods that act as a wallet, and enables the users to add a credit card to the wallet, and re-use in the future
# Before we start
In order to use instant payments, you need to set up the amount and currency.
mycheckWallet.setPayment({"currency":"USD","amount":"1.00"});
# How to get notified about a sucessfull transaction
Register to the afterAlternativePaymentComplete
callback event to know when the transaction been completed.
[Read more about callback events]
Returned event example:
{method: "SALE", status: "SUCCESS", transaction_id:"ALIPAY-Ir5JEqV0mcQiVg-1561985422"}
Not that the transaction on the user's end completed, you should verify, server to server the transaction ID, the complete users flow.
# Handling redirects
When using an alternative payment, the user will be redirected to the alternative provider and in the end of the payment process, back to the client. Upon redirecting back, we will send the transaction ID as mycheck-transaction-id query string.
Setting a return URL and a 3rd party callback URL:
mycheckWallet.setPayment({
"currency":"USD",
"amount":"1.00",
"return_url":"<current client's URL>",
"callback_url":"<3rd party's URL to be updated when the payment as succeeded/failed>"
});
Redirecting back handling example:
mycheckWallet.init("mywalletSdk", {
events: {
afterAlternativePaymentComplete: async (response) => {
try {
this.isAlternativePayment = true;
if (response && response.status === 'SUCCESS') {
// either use the transaction ID retreived from the query string or store it localy before you get redirected
const url = new URL(window.location);
const transactionId = url.searchParams.get('mycheck-transaction-id');
// go to confirmation page to end the flow
history.push('/payment-confirmation');
} else {
throw new Error();
}
} catch (err) {
this.buttonLoading = false;
const { error } = this.translations;
const errorMsg = !isEmptyObject(error) ? error.text : 'Something went wrong, please try later';
swal({
text: errorMsg,
button: 'Confirm',
});
}
},
},
});
# Triggering an alternative payment
Use the following function to trigger the alternative payment after setting its payment request parameters (using setPayment). This trigger will take care of the action required for this alternative payment such as redirect, display QR code, etc...
const token = await window.mycheckWallet.getCardToken();
# How to verify the transaction
To verify the transaction, please use the verifying transaction API.